FD Calculator implementation using Python
RD Calculator | |
Final Amount: | |
Total Interest: |
We will try to implement an FD calculator using Python.
Purpose of this tutorial is to get a better understanding of
python basics
and tkinter GUI library.
Also intended to those who are not familiar to programming, to develop an interest in Python.
You can see that how easy it is to work with Python.
Learning the basics of Python will surely help to automate many of the day-to-day tasks that you do on your computers.
Go through below sections, if you are interested in developing such tools by yourself.
Code Setup
Download and install the latest version of python from Python Website.
Keep your favorite Python editor ready. This can be a basic notepad application available by default for your OS or you can download and install any free editor like VS Code.
For GUI, we will have to use any third-party libraries. Lots of free options are available. For our FD calculator, we will use TKinter, which is easier to understand for a beginner.
Next, install TKinter using command pip install tk.
For this, open the command prompt by typing cmd in run window.
Navigate to the folder we want to place our code by typing the path like cd C:\Users\FD-Calculator
Then run the above installation command.
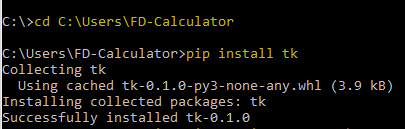
If you wish to get a quick tutorial of tkinter before starting with the sample, please have a look at the Tkinter Quick Reference.
Then create a file named fd-calculator.py in the same folder and copy the below codes step by step
or use the complete code at the end.
To run the code type python fd-calculator.py in the command prompt.
-
Import TKinter to your module
Import all options in Tkinter using below import command
Copiedfrom tkinter import * import tkinter as tk
-
Create main window
Following code creates main window, sets title and size. All the code should come inside this.
Copiedroot = tk.Tk() root.title("FD Calculator") root.geometry("300x200") #rest of code here root.mainloop()
-
Declare TKinter variables to hold principal, rate, months and frequency
CopiedintPr = tk.StringVar() intRate = tk.StringVar() intMon = tk.StringVar() intRes = tk.StringVar() intFreq = 4
-
Use Frames to align the input fields like a table
Create 3 frames and place 3 entries (text boxes) and its labels to hold the input values
CopiedfrPrincipal = Frame(root) frPrincipal.pack(fill=tk.X) lblPrincipal = Label(frPrincipal, text="Principal Amount") lblPrincipal.pack( side = LEFT) txtPrincipal = Entry(frPrincipal, textvariable=intPr, bd = 1) txtPrincipal.pack(side = RIGHT) frInterest = Frame(root) frInterest.pack(fill=tk.X) lblInterest = Label(frInterest, text="Interest Rate per Year") lblInterest.pack( side = LEFT) txtInterest = Entry(frInterest, textvariable=intRate, bd = 1) txtInterest.pack(side = RIGHT) frMonths = Frame(root) frMonths.pack(fill=tk.X) lblMonths = Label(frMonths, text="Number of Months") lblMonths.pack( side = LEFT) txtMonths = Entry(frMonths, textvariable=intMon, bd = 1) txtMonths.pack(side = RIGHT)
-
Place a button, with callback function calcFD() and align it at the bottom frame
CopiedtnCalc = tk.Button(root, text ="Calculate", command = calcFD) btnCalc.pack(side = BOTTOM)
-
Place a label to display the results
CopiedlblRes = tk.Label( root, text="" ) lblRes.pack()
-
Implement the calculation logic inside calcFD() function
Copieddef calcFD(): p = (intPr.get()) r = (intRate.get()) m = (intMon.get()) res = float(p) * ((1 + (float(r)/100) / intFreq) ** ((float(m)/12) * intFreq)) lblRes.config(text = "Final Amount is %d" % round(res)) return
Final Code
from tkinter import * import tkinter as tk root = tk.Tk() root.title("FD Calculator") root.geometry("300x200") intPr = tk.StringVar() intRate = tk.StringVar() intMon = tk.StringVar() intRes = tk.StringVar() intFreq = 4 def calcFD(): p = (intPr.get()) r = (intRate.get()) m = (intMon.get()) res = float(p) * ((1 + (float(r)/100) / intFreq) ** ((float(m)/12) * intFreq)) lblRes.config(text = "Final Amount is %d" % round(res)) return frPrincipal = Frame(root) frPrincipal.pack(fill=tk.X) lblPrincipal = Label(frPrincipal, text="Principal Amount") lblPrincipal.pack( side = LEFT) txtPrincipal = Entry(frPrincipal, textvariable=intPr, bd = 1) txtPrincipal.pack(side = RIGHT) frInterest = Frame(root) frInterest.pack(fill=tk.X) lblInterest = Label(frInterest, text="Interest Rate per Year") lblInterest.pack( side = LEFT) txtInterest = Entry(frInterest, textvariable=intRate, bd = 1) txtInterest.pack(side = RIGHT) frMonths = Frame(root) frMonths.pack(fill=tk.X) lblMonths = Label(frMonths, text="Number of Months") lblMonths.pack( side = LEFT) txtMonths = Entry(frMonths, textvariable=intMon, bd = 1) txtMonths.pack(side = RIGHT) btnCalc = tk.Button(root, text ="Calculate", command = calcFD) btnCalc.pack(side = BOTTOM) lblRes = tk.Label( root, text="" ) lblRes.pack() root.mainloop()
